Laravel send SMTP mail by Gmail
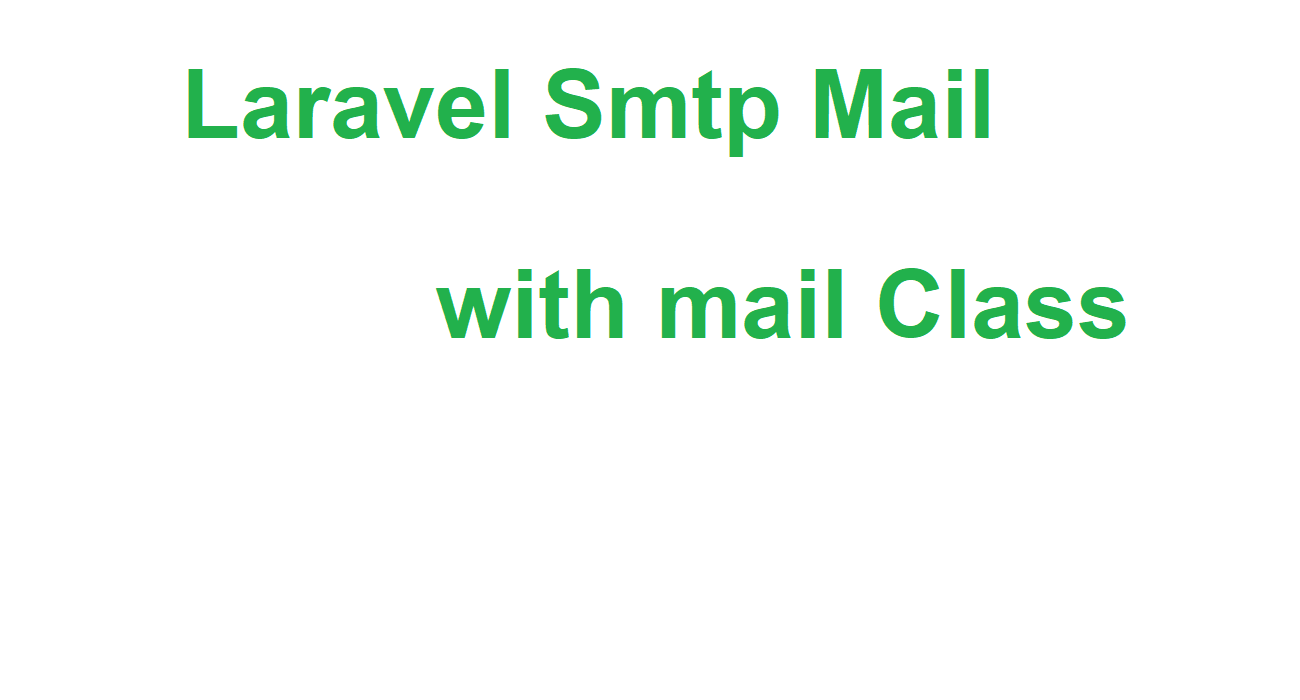
Laravel SMTP mail Send by Gmail (Mail Class) :
In Laravel, the Mail class and SMTP (Simple Mail Transfer Protocol) are integral parts of the framework's email functionality. Here's a brief explanation of each:
a. Mail Class:
- The Mail class in Laravel provides a simple and expressive API for sending emails from your application. It abstracts away the complexities of email sending and provides a clean interface for developers to work with.
- With the Mail class, you can easily send emails in various formats such as plain text, HTML, or Markdown. You can also attach files, embed images, and customize email headers.
- Laravel allows you to define mail templates using Markdown or Blade templates, making it easy to create consistent and visually appealing emails.
- The Mail class interacts with various drivers (such as SMTP, Mailgun, Sendmail, etc.) to send emails. You can configure the mail driver in your Laravel application's configuration files.
b. SMTP (Simple Mail Transfer Protocol):
- SMTP is a standard protocol used for sending emails over the Internet. It defines the rules and procedures for transferring email messages between email servers.
- In the context of Laravel, SMTP is one of the drivers used by the Mail class to send emails. When you configure Laravel to use SMTP, it connects to an SMTP server (such as Gmail's SMTP server) to deliver outgoing emails.
- SMTP servers typically require authentication (username and password) to send emails on behalf of a user or application. Laravel allows you to configure SMTP authentication credentials in your application's environment configuration (`.env` file).
- SMTP servers also use encryption protocols like SSL or TLS to secure the email transmission between the client (your Laravel application) and the server. Laravel allows you to specify the encryption method (TLS or SSL) and port number when configuring SMTP settings.
1) Setting up Gmail SMTP in Laravel:
- Instruct readers to update the .env file with Gmail SMTP settings:
Example :
MAIL_MAILER=smtp
MAIL_HOST=smtp.gmail.com
MAIL_PORT=465
MAIL_USERNAME=sndpbagg@gmail.com
MAIL_PASSWORD=vezknaguuqigzez
MAIL_ENCRYPTION=tls
MAIL_FROM_ADDRESS=sndpbagg@gmail.com
MAIL_FROM_NAME="${APP_NAME}"
2) Creating a Mail Class:
- Guide readers on creating a mail class using the php artisan make:mail command:
php artisan make:mail TestMail
- Show how to customize the email content and subject within the generated mail class (app/Mail/TestMail.php):
Make Route :
Route::POST('job_applications',[MailController ::class,'job_applied'])->name('job_applied');
3)Create Controller:
Create a new controller named MailController using the following command:
php artisan make:controller MailController
4) Define Controller Method:
Open the MailController located at app/Http/Controllers/MailController.php, and add a method named sendEmail:
import :
use Illuminate\Support\Facades\Mail;
use App\Mail\TestMail;
// notification Email wihen apply job application
$employer = User::where('id',$employer_id)->first();
$mailData = [
'job' => $job,
'user' => Auth::user(),
'employer'=>$employer
];
Mail::to($employer->email)->send(new TestMail($mailData));
5) Go To TestMail class and open (app→mail→TestMail)
here you can see 4 method -
- __construct() - The variable of the mail class that we are sending by the variable ($mailData) in the form of data array from the controller is being received here.
public $mailData;
public function __construct($mailData)
{
$this -> mailData = $mailData;
}
2.envelope() - here write email Subject
public function envelope()
{
return new Envelope(
subject: 'Job Notification Mail',
);
}
- content() - Here the view page is written view page will be sent by mail.
public function content()
{
return new Content(
view: 'email.job-notification-email', // this is view page path which is send by email
);
}
- attachments() - attechemnt file
Creating Email View: :
make a view page which is send by email and all details . view page name is job-notification-email.blade.php
<body>
<h2>Hello {{$mailData['employer']->name}}</h2>
<p>Title : {{$mailData['job']->title}}</p>
<p>Employee Details :</p>
<p>Name : {{$mailData['user']->name}} </p>
<p>Email : {{$mailData['user']->email}} </p>
<p>Mobile : {{$mailData['user']->mobile}} </p>
</body>
This setup allows you to send emails using Gmail SMTP in Laravel by leveraging the Mail class and configuring SMTP settings. The controller method triggers the email sending process, and the Mail class handles the creation and delivery of the email using the specified view file for the email content.

Sandipan Kr Bag
I'm a dedicated full-stack developer, entrepreneur, and the proud owner of ocec.org.in , hailing from the vibrant country of India. My passion lies in creating informative tutorials and sharing valuable tips that empower fellow artisans in their journey. With a deep-rooted love for technology, I've been an ardent enthusiast of PHP, Laravel, Angular, Vue, Node, JavaScript, jQuery, Codeigniter, and Bootstrap from their earliest days. My philosophy revolves around the values of hard work and unwavering consistency, driving me to continuously explore, create, and share my knowledge with the tech community.
* Hire MeRelated Posts
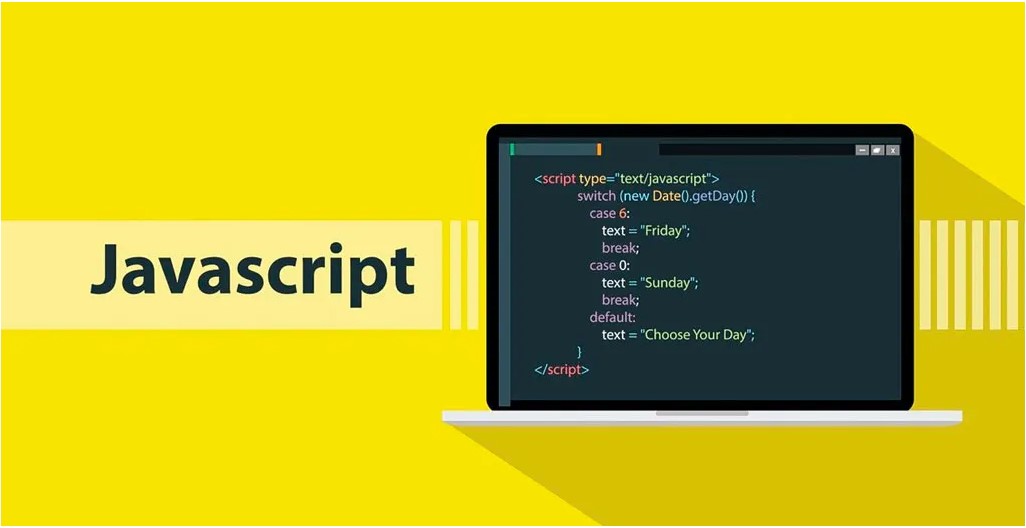
জাভাস্ক্রিপ্ট কি? এটি কেন ব্যবহার করা হয় ?
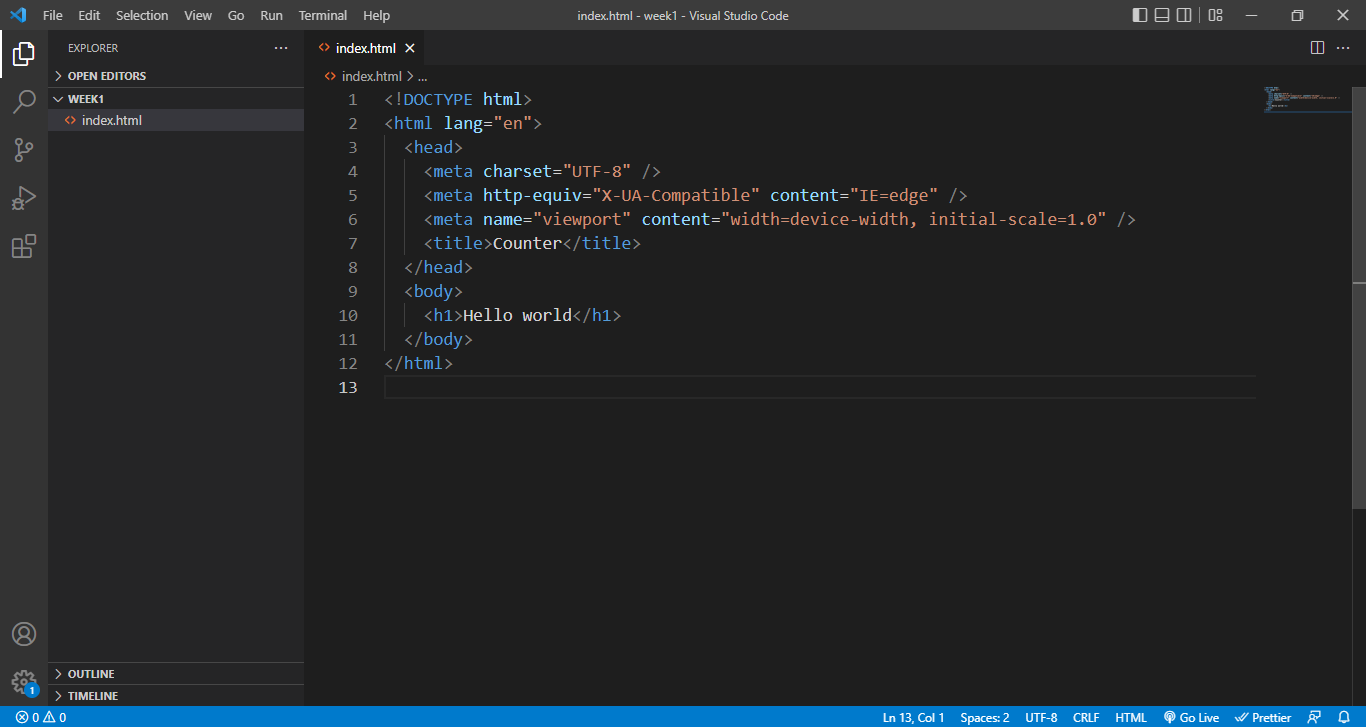
জাভাস্ক্রিপ্ট লেখার পদ্ধতি
Step-by-Step Guide a Dynamic Image Slider with HTML, CSS, and JavaScript
Search
Latest Posts
Using AOS (Animate On Scroll) in React with Tailwind CSS
3 months ago
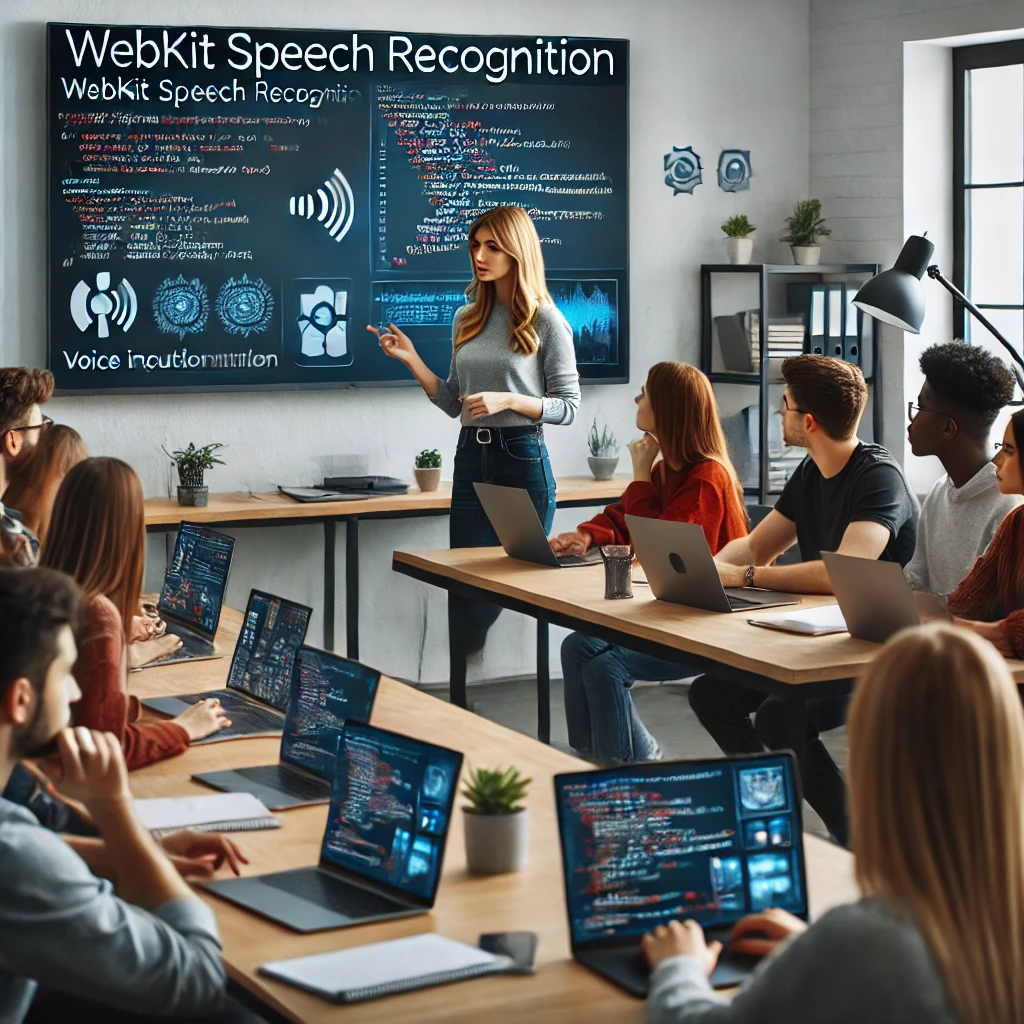
WebkitSpeechRecognition API
4 months ago
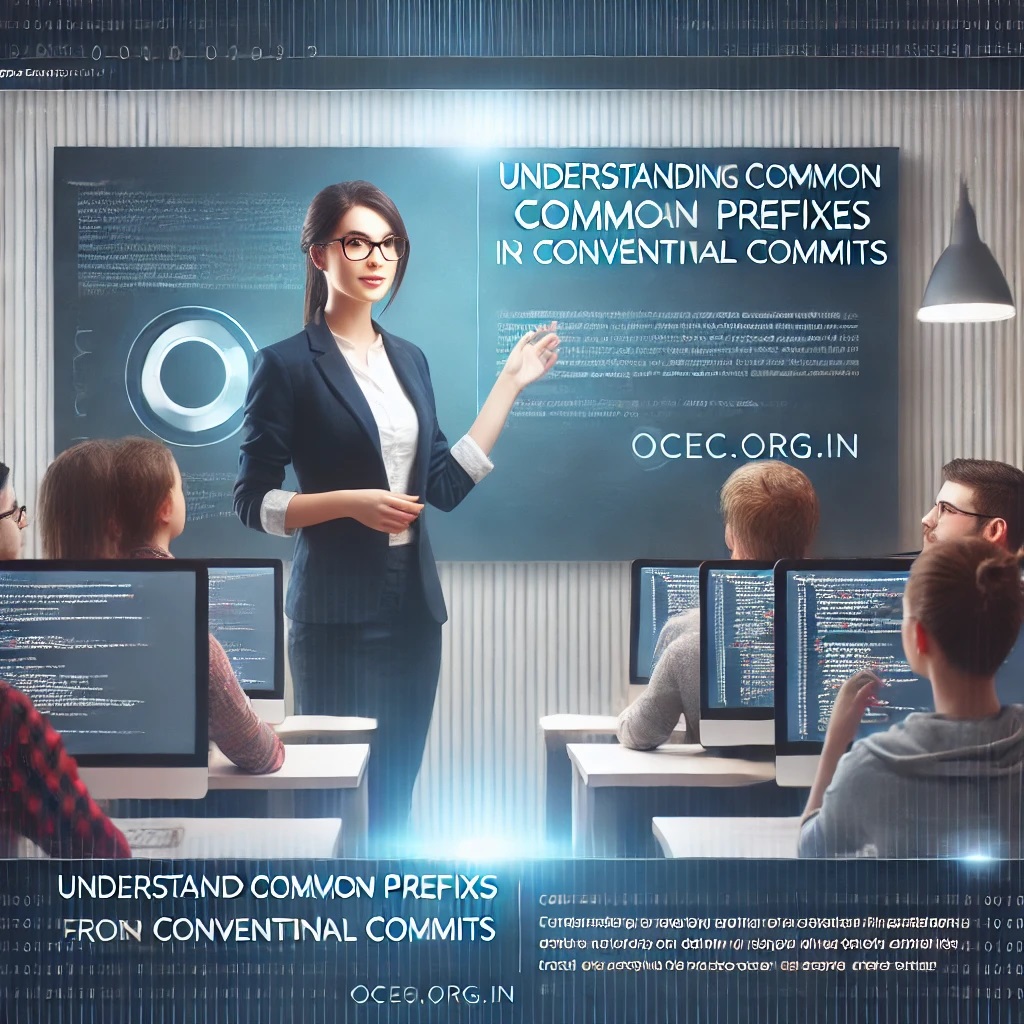
GitHub Understanding Common Prefixes in Conventional Commits
4 months ago
Subscribe to Our Newsletter
Get the latest updates straight to your inbox.