Create a Quiz Game with HTML, CSS, and JavaScript
Welcome to this comprehensive tutorial where we'll guide you through the process of creating an interactive quiz game using HTML, CSS, and JavaScript. Whether you're a beginner in web development or looking to enhance your coding skills, this step-by-step guide is designed to help you understand the fundamentals of frontend development.
In this tutorial, we'll cover essential aspects of building a quiz game, including structuring the HTML for questions, styling with CSS to create an appealing user interface, and implementing dynamic functionality with JavaScript. Follow along as we explore each step, providing detailed explanations and insights to ensure a thorough understanding of the concepts involved.
Code :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body {
font-family: Arial, sans-serif;
}
#quiz-container{
max-width: 600px;
margin:20px auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 8px;
text-align: center;
}
h1{
color:#333;
}
input{
padding: 8px;
margin: 10px 0;
}
button{
padding: 10px;
background-color: #4caf50;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
}
button:hover{
background-color: #45a049;
}
#result{
color:#f00;
font-weight: bold;
}
</style>
</head>
<body>
<div id="quiz-container">
<h1>Quiz Game</h1>
<div id="question-container"></div>
<input type="text" id="answer-input" placeholder="Type Your Answer">
<button onclick="submitAnswer()">Submit Answer</button>
<p id="result"></p>
</div>
<script>
let currentQuestion = 1;
let score = 0;
function startQuiz()
{
displayQuestion();
}
function displayQuestion()
{
const questionContainer = document.getElementById("question-container");
const answerInput = document.getElementById("answer-input");
const resultMessage = document.getElementById("result");
questionContainer.innerHTML = `<p>${getQuestion(currentQuestion)}</p>`; // here use tilled key
answerInput.value= ""; // blank value
resultMessage.innerHTML = ""; // blank value
// focus on the input field for user converience
answerInput.focus();
}
function submitAnswer()
{
const answerInput = document.getElementById("answer-input");
const userAnswer = answerInput.value.trim();
if(checkAnswer(currentQuestion,userAnswer))
{
document.getElementById("result").innerHTML = "correct!";
score++;
}else{
document.getElementById("result").innerHTML =
`wrong ! the correct Answer is: ${getCorrectAnswer(currentQuestion)}`;
}
currentQuestion++;
if(currentQuestion <=11)
{
displayQuestion();
}
else {
endquiz();
}
}
function endquiz(){
const quizContainer = document.getElementById("quiz-container");
quizContainer.innerHTML = `<h1>Quiz Completed ! Your final score is :
${score}/10</h1>`; // here use tilled key no single quotes
}
function getQuestion(number)
{
switch(number)
{
case 1 : return "What is the capital of France?";
case 2 : return "Which planet is known as the Red Planet?";
case 3 : return "What is the largest mammal in the world? ";
case 4 : return "What is the currency of Japan?";
case 5 : return "How many continents are there on Earth?";
case 6 : return "In which year did Christopher Columbus reach the Americas?";
case 7 : return "Who wrote 'Romeo and Juliet'?";
case 8 : return "What is the chemical symbol for gold?";
case 9 : return "Which element has the atomic number 1?";
case 10 : return "What is the largest ocean on Earth?";
case 11 : return "What is the capital of India?";
default : "invalid Question"; // this is optional
// you can add more questions here
}
}
function checkAnswer(number, answer)
{
switch(number)
{
case 1: return answer.toLowerCase() === "paris";
case 2: return answer.toLowerCase() === "mars";
case 3: return answer.toLowerCase() === "blue whale";
case 4: return answer.toLowerCase() === "yen";
case 5: return answer.toLowerCase() === "7";
case 6: return answer.toLowerCase() === "1492";
case 7: return answer.toLowerCase() === "william shakespeare";
case 8: return answer.toLowerCase() === "au";
case 9: return answer.toLowerCase() === "hydrogen";
case 10: return answer.toLowerCase() === "pacific";
case 11: return answer.toLowerCase() === "new delhi";
default: return false;
}
}
function getCorrectAnswer(number)
{
switch(number)
{
case 1 : return "paris";
case 2 : return "mars";
case 3 : return "blue whale";
case 4 : return "yen";
case 5 : return "7";
case 6 : return "1492";
case 7 : return "william shakespeare";
case 8 : return "au";
case 9 : return "hydrogen";
case 10 : return "pacific";
case 11 : return "new delhi";
default: return "Invalid Question";
}
}
// start the quiz
startQuiz();
</script>
</body>
</html>

Sandipan Kr Bag
I'm a dedicated full-stack developer, entrepreneur, and the proud owner of ocec.org.in , hailing from the vibrant country of India. My passion lies in creating informative tutorials and sharing valuable tips that empower fellow artisans in their journey. With a deep-rooted love for technology, I've been an ardent enthusiast of PHP, Laravel, Angular, Vue, Node, JavaScript, jQuery, Codeigniter, and Bootstrap from their earliest days. My philosophy revolves around the values of hard work and unwavering consistency, driving me to continuously explore, create, and share my knowledge with the tech community.
* Hire MeRelated Posts
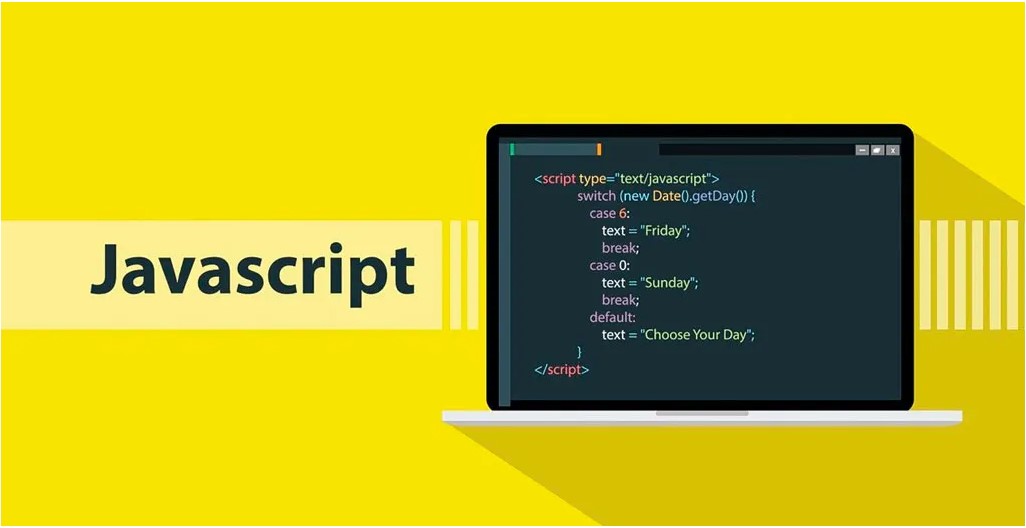
জাভাস্ক্রিপ্ট কি? এটি কেন ব্যবহার করা হয় ?
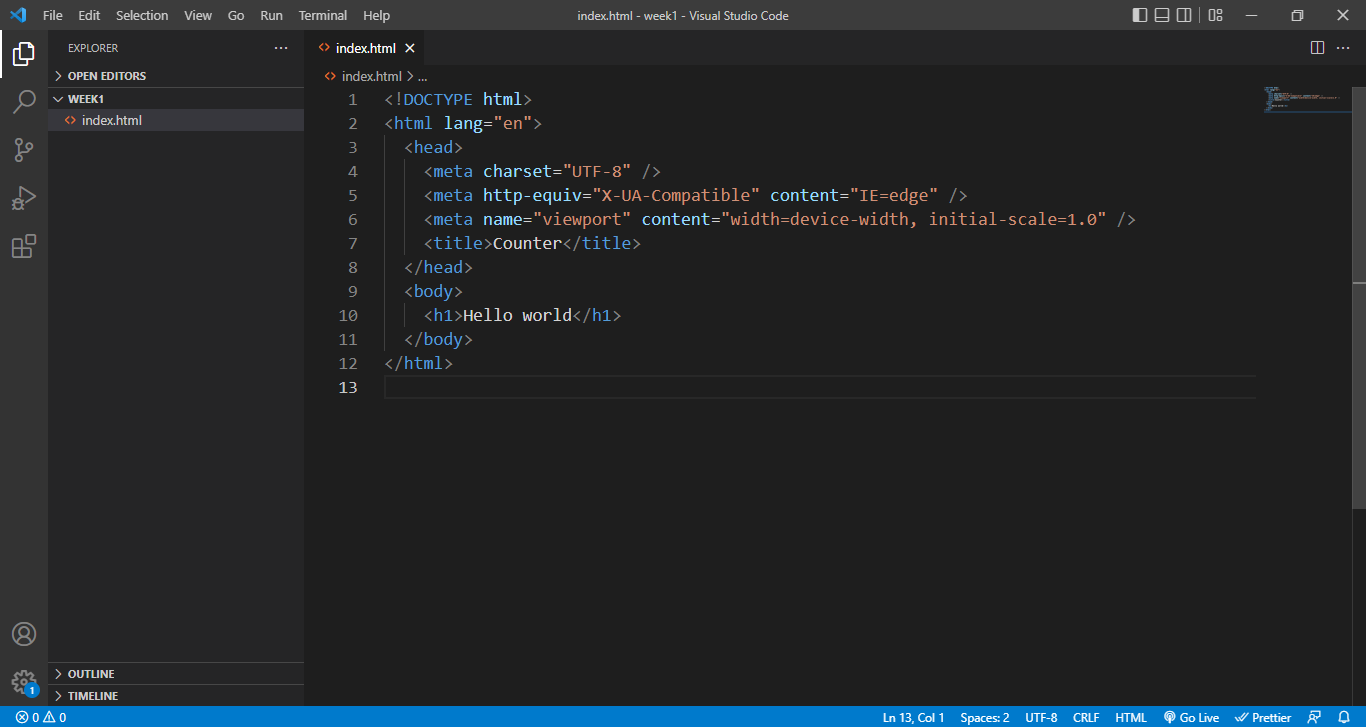
জাভাস্ক্রিপ্ট লেখার পদ্ধতি
Step-by-Step Guide a Dynamic Image Slider with HTML, CSS, and JavaScript
Search
Latest Posts
Using AOS (Animate On Scroll) in React with Tailwind CSS
1 month ago
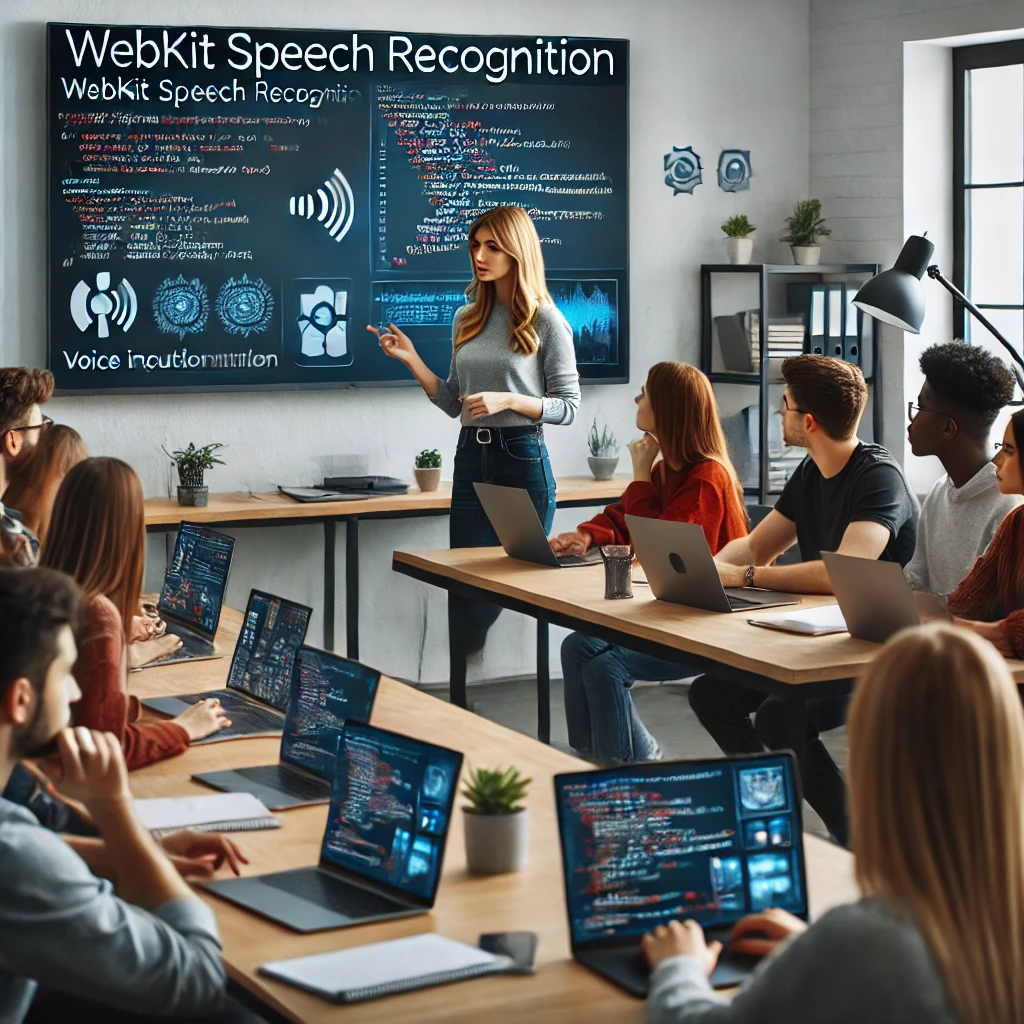
WebkitSpeechRecognition API
2 months ago
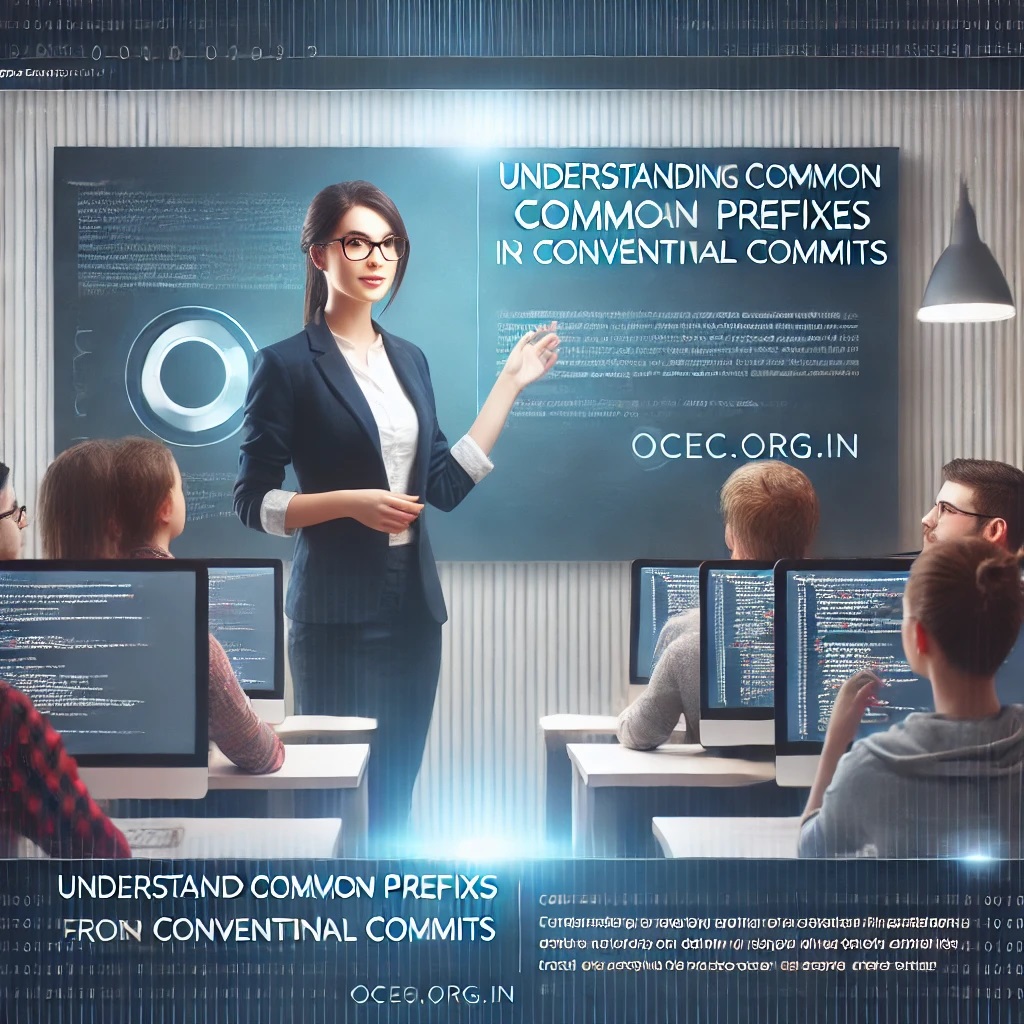
GitHub Understanding Common Prefixes in Conventional Commits
2 months ago
Subscribe to Our Newsletter
Get the latest updates straight to your inbox.